Warning: main(/www/www/htdocs/style/globals.php) [function.main]: failed to open stream: No such file or directory in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 1
Warning: main() [function.include]: Failed opening '/www/www/htdocs/style/globals.php' for inclusion (include_path='.:/www/www/common:/www/www/php/lib/php') in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 1
Warning: main(/www/www/htdocs/style/header.php) [function.main]: failed to open stream: No such file or directory in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 8
Warning: main() [function.include]: Failed opening '/www/www/htdocs/style/header.php' for inclusion (include_path='.:/www/www/common:/www/www/php/lib/php') in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 8
Create and execute a new child process, given a relative path
#include <spawn.h>
pid_t spawnp( const char * file,
int fd_count,
const int fd_map[ ],
const struct inheritance * inherit,
char * const argv[ ],
char * const envp[ ] );
- file
- If this argument contains a slash, it's used as the pathname of the
executable; otherwise, the PATH environment
variable is searched for file.
- fd_count
- The number of entries in the fd_map array.
- fd_map
- An array of file descriptors that you want the child process to inherit.
If fd_count isn't 0, fd_map must contain
at least fd_count file descriptors, up to OPEN_MAX
FDs.
The first three entries (if specified) become the child process's
standard input, standard output, and standard error
(stdin, stdout, and stderr).
If fd_count is 0, fd_map is ignored.
If you set fdmap[X] to
SPAWN_FDCLOSED instead of to a
valid file descriptor, the file descriptor X is closed in the
child process.
If fd_count is 0, the child process inherits all file descriptors
(except for the ones modified with fcntl()'s
FD_CLOEXEC flag).
- inherit
- A structure, of type struct inheritance, that indicates
what you want the child process to inherit from the parent.
For more information, see
“inheritance structure,”
in the documentation for spawn().
 |
If you want to
spawnp()
remotely, set the nd member of the inheritance structure to the
node descriptor. See the
netmgr_strtond()
function. |
- argv
- A pointer to an argument vector.
The value in argv[0] should represent the filename of the
program being
loaded, but can be NULL if no arguments are being passed.
The last member of argv must be a NULL
pointer.
The value of argv can't be NULL.
- envp
- A pointer to an array of character
pointers, each pointing to a string defining an environment variable.
The array is terminated with a NULL pointer.
Each pointer points to a character string of the form:
variable=value
that's used to define an environment variable.
If the value of envp is NULL, then the child
process inherits the environment of the parent process.
libc
Use the -l c option to
qcc
to link against this library.
This library is usually included automatically.
The spawnp() function creates and executes a new child process, named in file.
It sets the SPAWN_CHECK_SCRIPT and
SPAWN_SEARCH_PATH flags, and then calls
spawn().
 |
If the new child process is a shell script, the first line must start with
#!, followed by the path of the program to run
to interpret the script, optionally followed by one argument.
The script must also be marked as executable.
For more information, see
“The first line”
in the Writing Shell Scripts chapter of the
Neutrino User's Guide. |
The spawnp() function is a QNX function (based on the POSIX 1003.1d draft standard).
The C library also includes several specialized spawn*() functions.
Their names consist of spawn followed by several letters:
This suffix:
|
Indicates the function takes these arguments:
|
e
|
An array of environment variables.
|
l
|
A NULL-terminated list of arguments to the program.
|
p
|
A relative path. If the path doesn't contain a slash, the
PATH environment variable is searched for the program.
This suffix also lets the #! construction work; see
SPAWN_CHECK_SCRIPT in the documentation for
spawn().
|
v
|
A vector of arguments to the program. |
To view the documentation for a function, click its name in this diagram:
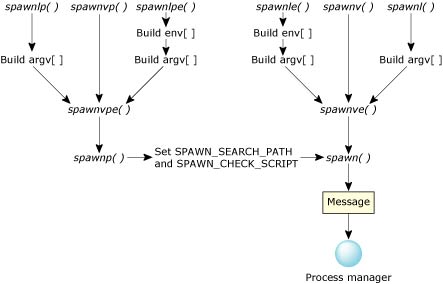
Most of the spawn*() functions do a lot of work before a message is sent to
procnto.
The child process inherits the following attributes of the parent process:
- process group ID (unless SPAWN_SETGROUP is set in inherit.flags)
- session membership
- real user ID and real group ID
- effective user ID and effective group ID
- supplementary group IDs
- priority and scheduling policy
- current working directory and root directory
- file-creation mask
- signal mask (unless SPAWN_SETSIGMASK is set in inherit.flags)
- signal actions specified as SIG_DFL
- signal actions specified as SIG_IGN (except the
ones modified by inherit.sigdefault when
SPAWN_SETSIGDEF is set in inherit.flags).
The child process has several differences from the parent process:
- Signals set to be caught by the parent process are set to the
default action (SIG_DFL).
- The child process's tms_utime, tms_stime,
tms_cutime, and tms_cstime are tracked separately
from the parent's.
- The number of seconds left until a SIGALRM signal
would be generated is set to zero for the child process.
- The set of pending signals for the child process is empty.
- File locks set by the parent aren't inherited.
- Per-process timers created by the parent aren't inherited.
- Memory locks and mappings set by the parent aren't inherited.
If the child process is spawned on a remote node, the process group ID and
the session membership aren't set;
the child process is put into a new session and a new process group.
The child process can access its environment by using the
environ
global variable (found in <unistd.h>).
If the file is on a filesystem mounted with the ST_NOSUID flag set,
the effective user ID, effective group ID, saved set-user ID and saved set-group ID are unchanged for the child process.
Otherwise, if the set-user ID mode bit is set, the effective user ID of the child process is set
to the owner ID of file.
Similarly, if the set-group ID mode bit is set, the effective group ID of the child process
is set to the group ID of file.
The real user ID, real group ID and supplementary group IDs of the child process remain the same as
those of the parent process.
The effective user ID and effective group ID of the child process are saved as the saved set-user ID and
the saved set-group ID used by the
setuid().
Suppose you call spawnp() like this:
spawnp(file, fd_count, fd_map, inherit, argv, envp);
The spawnp() function does the following:
- creates an empty attribute if inherit is NULL
- sets the CHECK_SCRIPT flag
- sets the SPAWN_SEARCH_PATH flag
and then calls spawn().
 |
A parent/child relationship doesn't imply that the child process dies when the parent process dies. |
The process ID of the child process, or -1 if an error occurs
(errno is set).
 |
If you set SPAWN_EXEC in the flags member of
the inheritence structure, spawnp() doesn't return,
unless an error occurred. |
- E2BIG
- The number of bytes used by the argument list and environment list of the new child process
is greater than ARG_MAX bytes.
- EACCESS
- Search permission is denied for a directory listed in the path prefix
of the new child process or the new child process's file doesn't have the execute bit set
or file's filesystem was mounted with the ST_NOEXEC flag.
- EAGAIN
- Insufficient resources available to create the child process.
- EBADF
- An entry in fd_map refers to an invalid file descriptor, or
an error occurred duplicating open file descriptors to the new process.
- EFAULT
- One of the buffers specified in the function call is invalid.
- ELOOP
- Too many levels of symbolic links or prefixes.
- EMFILE
- Insufficient resources available to load the new executable
image or to remap file descriptors in the child process.
- ENAMETOOLONG
- The length of file exceeds PATH_MAX or a
pathname component is longer than NAME_MAX.
- ENOENT
- The file identified by the file argument is empty,
or one or more components of the pathname of the child process don't exist.
- ENOEXEC
- The child process's file has the correct permissions, but isn't in the correct format for an executable.
- ENOMEM
- Insufficient memory available to create the child process.
- ENOSYS
- The spawnp() function isn't implemented for the filesystem specified in file.
- ENOTDIR
- A component of the path prefix of the child process isn't a directory.
QNX Neutrino
Safety: | |
Cancellation point |
No |
Interrupt handler |
No |
Signal handler |
No |
Thread |
Yes |
execl(),
execle(),
execlp(),
execlpe(),
execv(),
execve(),
execvp(),
execvpe(),
getenv(),
netmgr_strtond(),
putenv(),
setenv(),
sigaddset(),
sigdelset(),
sigemptyset(),
sigfillset(),
spawn(),
spawnl(),
spawnle(),
spawnlp(),
spawnlpe(),
spawnv(),
spawnve(),
spawnvp(),
spawnvpe(),
wait(),
waitpid()
Processes and Threads
chapter of Getting Started with QNX Neutrino
Warning: main(/www/www/htdocs/style/footer.php) [function.main]: failed to open stream: No such file or directory in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 554
Warning: main() [function.include]: Failed opening '/www/www/htdocs/style/footer.php' for inclusion (include_path='.:/www/www/common:/www/www/php/lib/php') in /www/www/docs/6.4.1/neutrino/lib_ref/s/spawnp.html on line 554